mirror of
https://github.com/cloudflare/cloudflared.git
synced 2025-05-11 23:16:35 +00:00
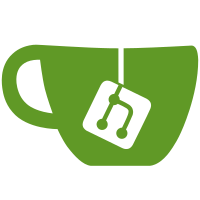
Going forward, the only protocols supported will be QUIC and HTTP2, defaulting to QUIC for "auto". Selecting h2mux protocol will be forcibly upgraded to http2 internally.
53 lines
1.4 KiB
Go
53 lines
1.4 KiB
Go
package edgediscovery
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"net"
|
|
"strings"
|
|
)
|
|
|
|
const (
|
|
protocolRecord = "protocol-v2.argotunnel.com"
|
|
)
|
|
|
|
var (
|
|
errNoProtocolRecord = fmt.Errorf("No TXT record found for %s to determine connection protocol", protocolRecord)
|
|
)
|
|
|
|
type PercentageFetcher func() (ProtocolPercents, error)
|
|
|
|
// ProtocolPercent represents a single Protocol Percentage combination.
|
|
type ProtocolPercent struct {
|
|
Protocol string `json:"protocol"`
|
|
Percentage int32 `json:"percentage"`
|
|
}
|
|
|
|
// ProtocolPercents represents the preferred distribution ratio of protocols when protocol isn't specified.
|
|
type ProtocolPercents []ProtocolPercent
|
|
|
|
// GetPercentage returns the threshold percentage of a single protocol.
|
|
func (p ProtocolPercents) GetPercentage(protocol string) int32 {
|
|
for _, protocolPercent := range p {
|
|
if strings.ToLower(protocolPercent.Protocol) == strings.ToLower(protocol) {
|
|
return protocolPercent.Percentage
|
|
}
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// ProtocolPercentage returns the ratio of protocols and a specification ratio for their selection.
|
|
func ProtocolPercentage() (ProtocolPercents, error) {
|
|
records, err := net.LookupTXT(protocolRecord)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if len(records) == 0 {
|
|
return nil, errNoProtocolRecord
|
|
}
|
|
|
|
var protocolsWithPercent ProtocolPercents
|
|
err = json.Unmarshal([]byte(records[0]), &protocolsWithPercent)
|
|
return protocolsWithPercent, err
|
|
}
|