mirror of
https://github.com/cloudflare/cloudflared.git
synced 2025-05-11 06:06:35 +00:00
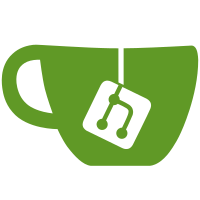
This commit makes cloudflared use the API token provided during login instead of service key. In addition, it eliminates some of the old formats since those are legacy and we only support cloudflared versions newer than 6 months.
52 lines
1.5 KiB
Go
52 lines
1.5 KiB
Go
package certutil
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestLoadOriginCert(t *testing.T) {
|
|
cert, err := DecodeOriginCert([]byte{})
|
|
assert.Equal(t, fmt.Errorf("Cannot decode empty certificate"), err)
|
|
assert.Nil(t, cert)
|
|
|
|
blocks, err := ioutil.ReadFile("test-cert-unknown-block.pem")
|
|
assert.Nil(t, err)
|
|
cert, err = DecodeOriginCert(blocks)
|
|
assert.Equal(t, fmt.Errorf("Unknown block RSA PRIVATE KEY in the certificate"), err)
|
|
assert.Nil(t, cert)
|
|
}
|
|
|
|
func TestJSONArgoTunnelTokenEmpty(t *testing.T) {
|
|
cert, err := DecodeOriginCert([]byte{})
|
|
blocks, err := ioutil.ReadFile("test-cert-no-token.pem")
|
|
assert.Nil(t, err)
|
|
cert, err = DecodeOriginCert(blocks)
|
|
assert.Equal(t, fmt.Errorf("Missing token in the certificate"), err)
|
|
assert.Nil(t, cert)
|
|
}
|
|
|
|
func TestJSONArgoTunnelToken(t *testing.T) {
|
|
// The given cert's Argo Tunnel Token was generated by base64 encoding this JSON:
|
|
// {
|
|
// "zoneID": "7b0a4d77dfb881c1a3b7d61ea9443e19",
|
|
// "apiToken": "test-service-key",
|
|
// "accountID": "abcdabcdabcdabcd1234567890abcdef"
|
|
// }
|
|
CloudflareTunnelTokenTest(t, "test-cloudflare-tunnel-cert-json.pem")
|
|
}
|
|
|
|
func CloudflareTunnelTokenTest(t *testing.T, path string) {
|
|
blocks, err := ioutil.ReadFile(path)
|
|
assert.Nil(t, err)
|
|
cert, err := DecodeOriginCert(blocks)
|
|
assert.Nil(t, err)
|
|
assert.NotNil(t, cert)
|
|
assert.Equal(t, "7b0a4d77dfb881c1a3b7d61ea9443e19", cert.ZoneID)
|
|
key := "test-service-key"
|
|
assert.Equal(t, key, cert.APIToken)
|
|
}
|